迭代器
迭代器模式提供一种方法顺序访问一个聚合对象中的各个元素,而又不暴露其内部的表示。
适用性
- 访问一个聚合对象的内容而无需暴露它的内部表示。
- 支持对聚合对象的多种遍历。
- 为遍历不同的聚合结构提供一个统一的接口(即,支持多态迭代)。
结构
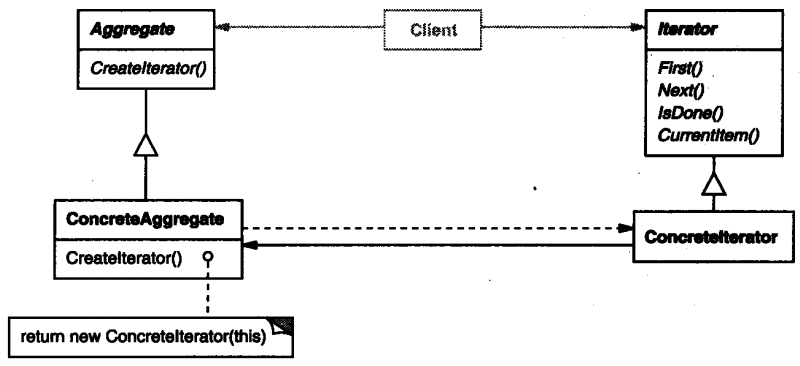
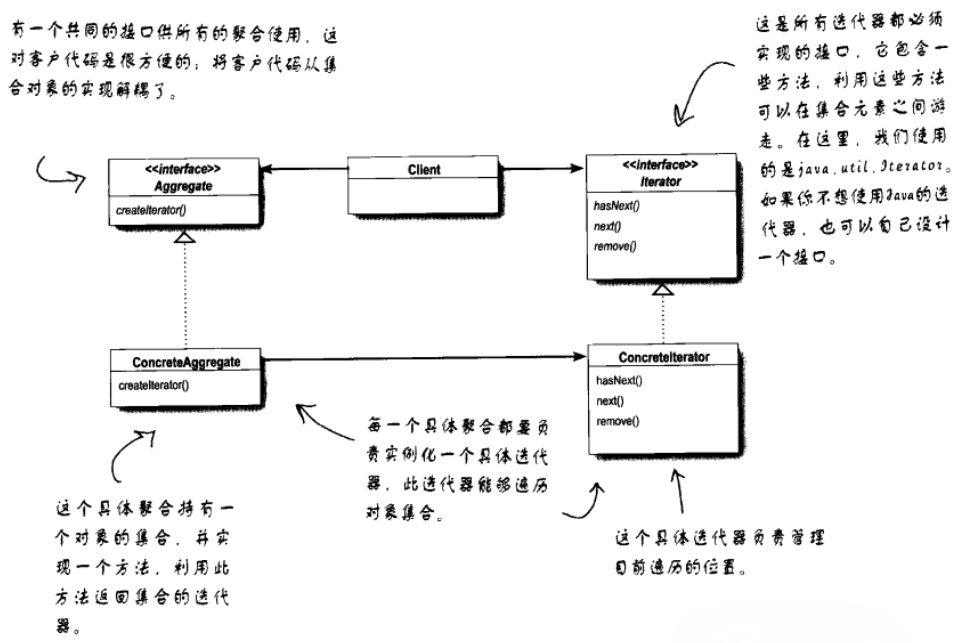
Iterator - 迭代器定义访问和遍历元素的接口。
ConcreteIterator - 具体迭代器实现迭代器接口。对该聚合遍历时跟踪当前位置。
Aggregate - 聚合定义创建相应迭代器对象的接口。
ConcreteAggregate - 具体聚合实现创建相应迭代器的接口,该操作返回ConcreteIterator的一个适当的实例。
协作:
ConcreteIterator跟踪聚合中的当前对象,并能够计算出待遍历的后继对象。
示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105
| #include <iostream> template <typename Item> class ConcreteAggregate;
template <typename Item> class Iterator { public: virtual void first() = 0; virtual void next() = 0; virtual bool is_done() const = 0; virtual Item& current_item() = 0; };
template <typename Item> class Aggregate { public: virtual Iterator<Item>* create_iterator() = 0; virtual long count() const = 0; virtual Item& get(long index) = 0; };
template <typename Item> class ConcreteIterator : public Iterator<Item> { public: ConcreteIterator(ConcreteAggregate<Item>* aAggregate); virtual void first(); virtual void next(); virtual bool is_done() const; virtual Item& current_item(); protected: ConcreteAggregate<Item>* m_aggregate; long m_current; };
template <typename Item> class ConcreteAggregate : public Aggregate<Item> { public: virtual Iterator<Item>* create_iterator(); virtual long count() const {return 5;} virtual Item& get(long index) { if (index > 4) throw; return m_array[index]; } protected: Item m_array[5]; };
template <typename Item> ConcreteIterator<Item>::ConcreteIterator( ConcreteAggregate<Item>* aAggregate ):m_aggregate(aAggregate), m_current(0) {} template <typename Item> void ConcreteIterator<Item>::first() { m_current = 0; } template <typename Item> void ConcreteIterator<Item>::next() { ++m_current; } template <typename Item> bool ConcreteIterator<Item>::is_done() const { return m_current >= m_aggregate->count(); } template <typename Item> Item& ConcreteIterator<Item>::current_item() { if (is_done()) { std::cout << "Iterator Out of Bounds" << std::endl; throw; } return m_aggregate->get(m_current); }
template <typename Item> Iterator<Item>* ConcreteAggregate<Item>::create_iterator() { return new ConcreteIterator<Item>(this); }
int main() { Aggregate<char>* numbers = new ConcreteAggregate<char>(); Iterator<char>* it = numbers->create_iterator(); char index('a'); for (it->first(); !it->is_done(); it->next()) { it->current_item() = index; ++index; } for (it->first(); !it->is_done(); it->next()) { std::cout << it->current_item() << " "; } std::cout << std::endl; system("Pause"); }
|
相关模式
- Composite: 迭代器常被应用到像复合这样的递归结构上。
- Factory Method: 多态迭代器靠Factory Method来例化适当的迭代器子类。
- Memento: 常与迭代器模式一起使用。迭代器可使用一个memento来捕获一个迭代的状态。迭代器在其内部存储memento。
[1] 设计模式:可复用面向对象软件的基础
[2] Head First设计模式